Videocall
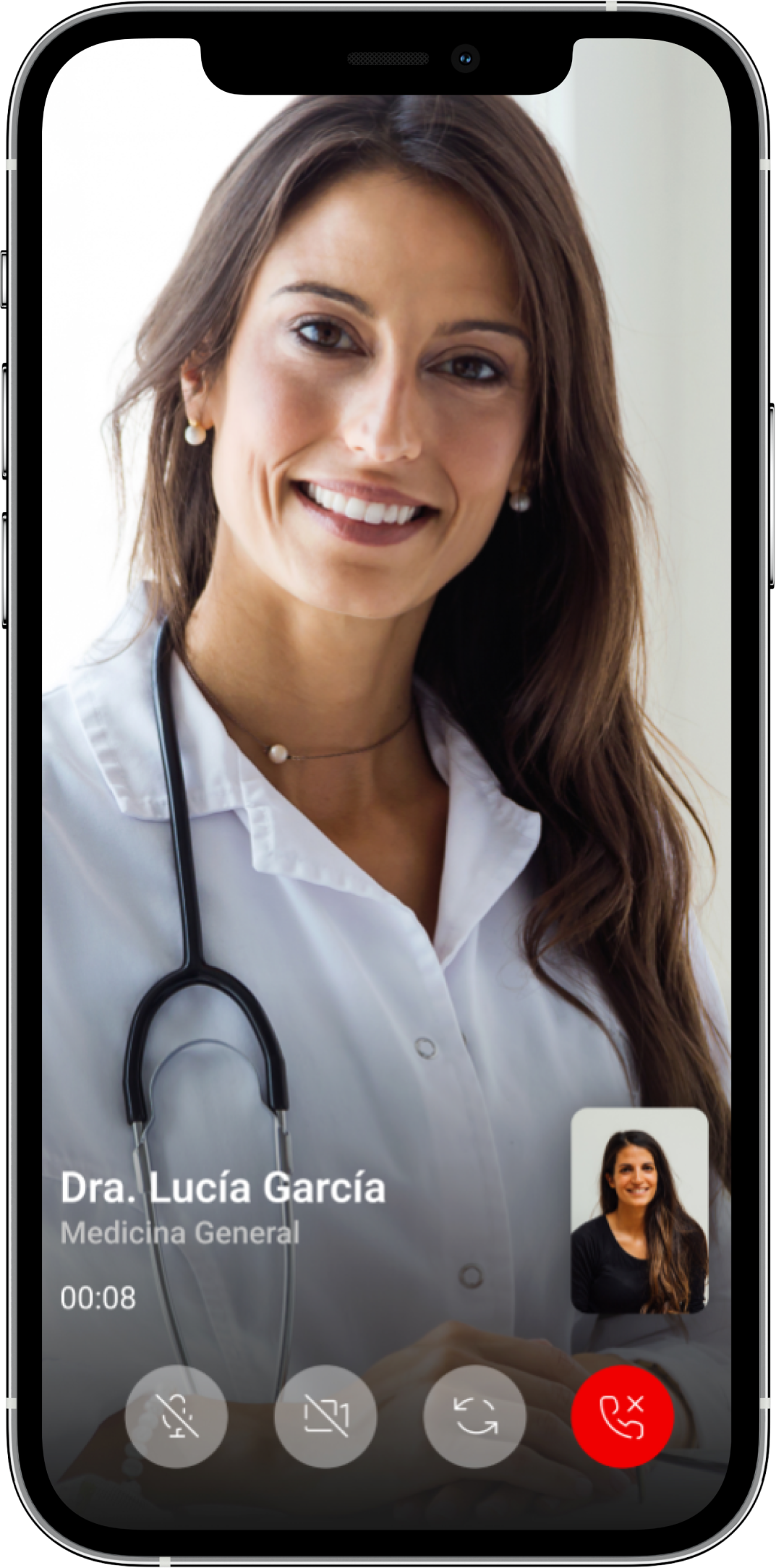
Videocall
Once we initialized the SDK and authenticated the user, we can retrieve the MeetingDoctors UI using the following method:
parameters
- origin (default nil)
- The origin viewController from which the videocall will be presented.
- animated (default true)
- If the presentation of the view will be animated.
errors
- Framework not initializated or user not authenticated
MeetingDoctorsError.illegalStateException(reason: .frameworkInitializationFailed))
- Installation not created
MeetingDoctorsError.illegalStateException(reason: .installationNotCreated))
- Feature not enabled for that user
MeetingDoctorsError.videoCall(reason: .videoCallForbidden))
public static func startVideocall(
origin: UIViewController? = nil,
animated: Bool = true,
completion: @escaping @Sendable (Swift.Result<Void, any Error>) -> Void
)
Implementation example
So we can invoke the videocall by adding the following example:
MeetingDoctors.startVideocall(origin: self) { result in
switch result {
case let .success(value):
// Handle success
case let .failure(error):
// Handle error
}
}
Top divider
In the banner you can subscribe to the following notifications to know the status of the videocall:
private func bindNotifications() {
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocallPickUp),
name: NSNotification.Name.MeetingDoctors.VideoCall.PickedUp,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocallFinished),
name: NSNotification.Name.MeetingDoctors.VideoCall.Cancelled,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocallFinished),
name: NSNotification.Name.MeetingDoctors.VideoCall.Finished,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.didBecomeActive),
name: UIApplication.didBecomeActiveNotification,
object: nil)
}
- The following method has been exposed to know if the user has any videocall started:
@objc func didBecomeActive() {
MeetingDoctors.hasAnyVideocallStarted { [weak self] value in
DispatchQueue.main.async {
value ? self?.videocallPickUp() : self?.videocallFinished()
}
}
}
Notifications
- The following notifications have been added in order to obtain more information on the status of the different points of the process:
private func bindNotifications() {
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_requested,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_cancelled_user,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_professional_ready,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_cancelled_time_expired,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_user_joined,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_finished,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_cancelled_professional_busy,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_error_network,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_error_system,
object: nil)
NotificationCenter
.default
.addObserver(self,
selector: #selector(self.videocall(notification:)),
name: NSNotification.Name.MeetingDoctors.VideoCall.videocall_cancelled_user_requested_new,
object: nil)
}
@objc func videocall(notification: Notification) {
let item = notification.userInfo?[Notification.Key.VideoCall.Item] as? MDVCExposedMethodsModel
print("[Notification] videocall: \(String(describing: item))")
}
- The the content of struct
MDVCExposedMethodsModel
is:
public struct MDVCExposedMethodsModel {
var videoconsultation_id: Int?
var videocall_id: String?
var videoconsultation_type: String?
var videoconsultation_specialty: String?
var customer_token: String?
var professional_name: String?
var professional_token: String?
var timestamp: Date?
var customer_cancellation_argument: String?
}