Professional List
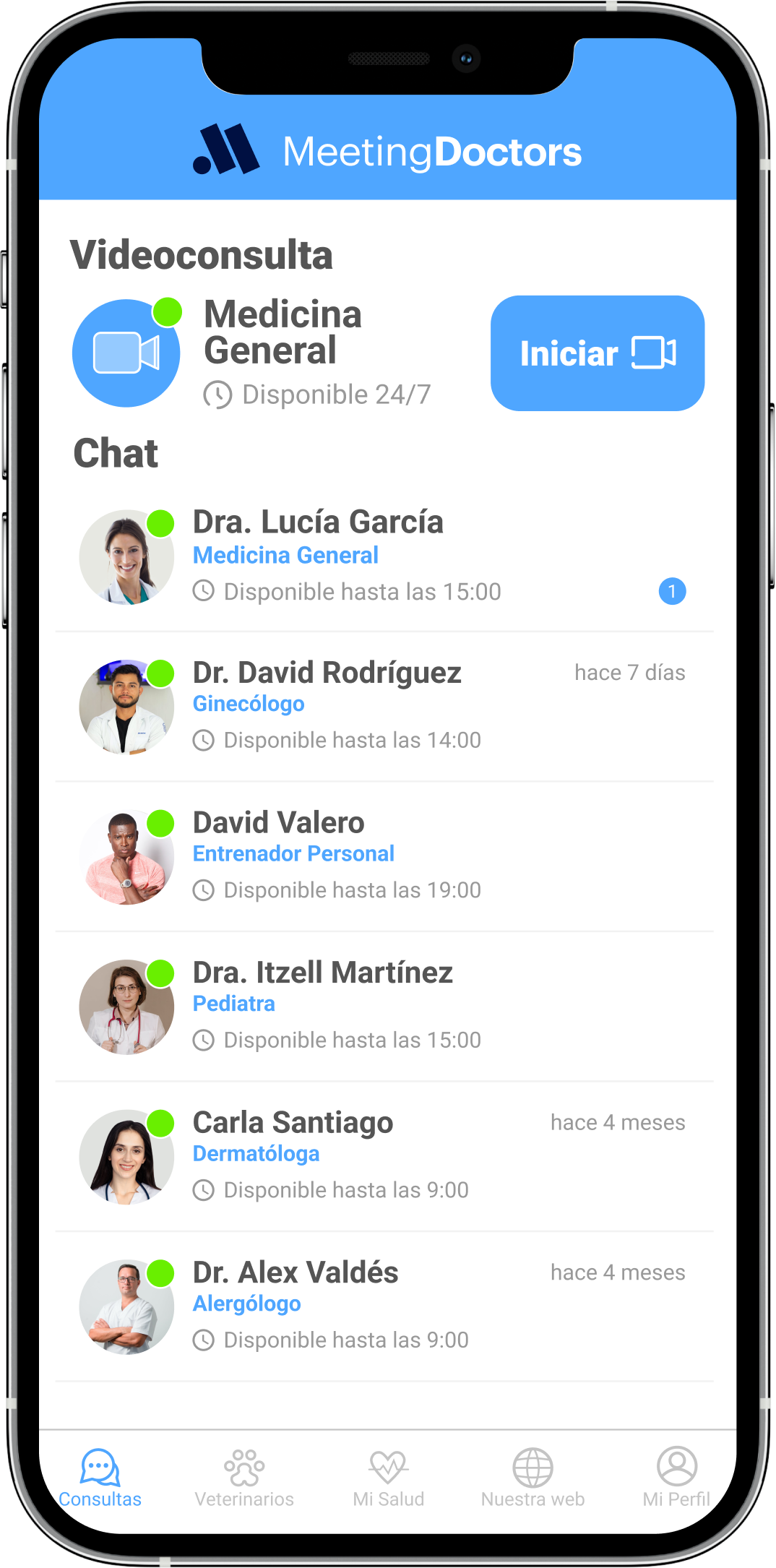
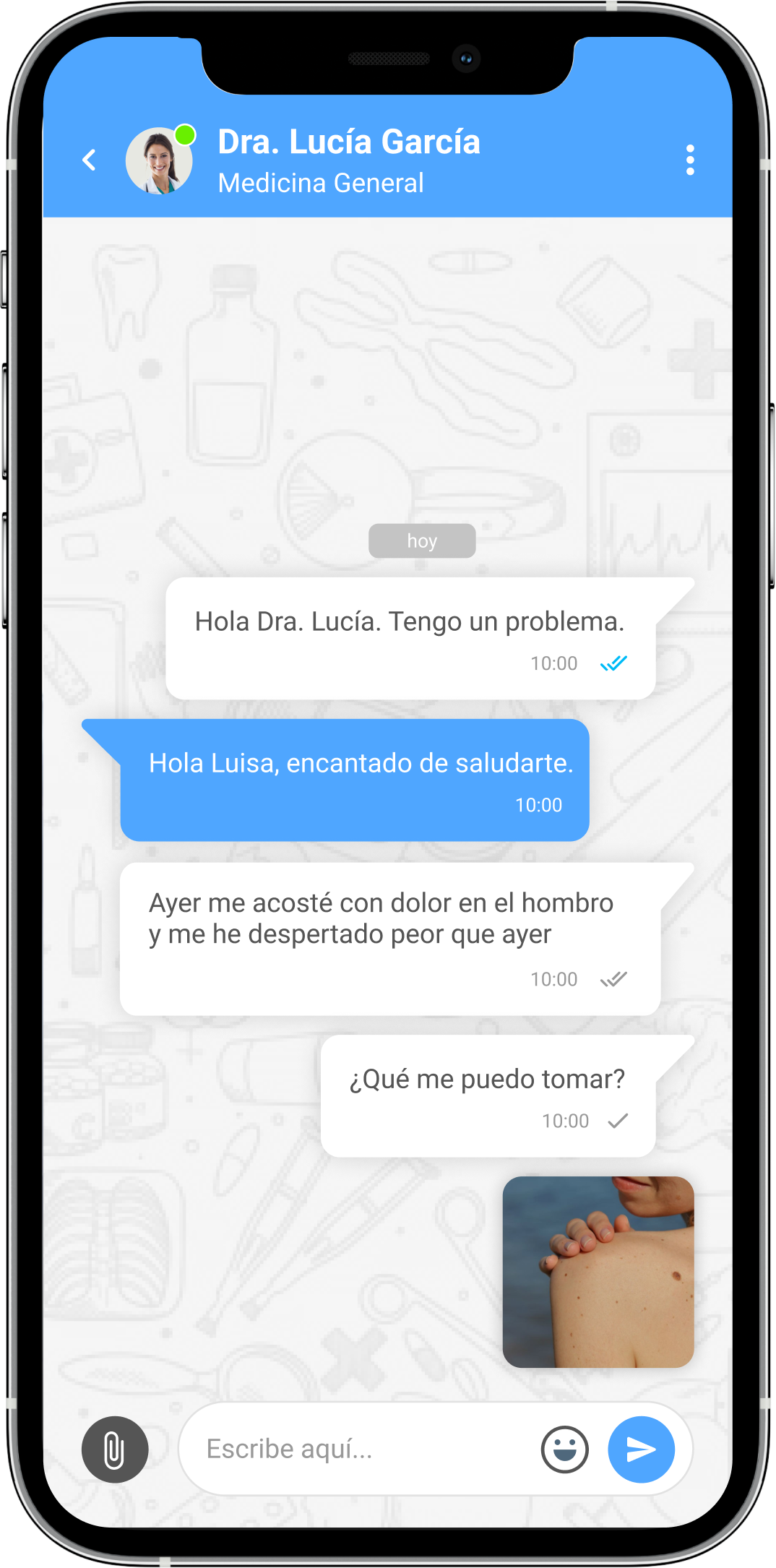
Professional List ViewController
Once we initialized the SDK and authenticated the user, we can retrieve the MeetingDoctors UI using the following method:
final public class func professionalListViewController(with filter: MeetingDoctorsSDK.MeetingDoctorsFilterType = MeetingDoctorsFilter.default, showHeader: Bool = false, divider: (MeetingDoctorsSDK.MeetingDoctorsDividerType)? = nil, topDividers: [MeetingDoctorsSDK.MeetingDoctorsDividerType]? = [], onUpdateLayout listener: ((CGSize) -> Void)? = nil) -> MeetingDoctorsSDK.MeetingDoctorsResult<UIViewController>
Method parameters have been overloaded with default values. So we can invoke the list of doctors by adding the following call:
[...]
let result = MeetingDoctors.professionalListViewController()
if let wrapController = result.value {
// do some stuff
}
[...]
Filtered contact list
By default, we show all professionals configured.
In order to filter the list of contacts, we must use the previous function passing a MeetingDoctorsFilter:
MeetingDoctors.messengerViewController(with: MeetingDoctorsFilterType, onUpdateLayout: ((CGSize) -> Void)?)
It takes as a parameter the filters wanted to be applied and optionally a size in case we need to update a height constraint to fit the result list.
The next included example shows how we could filter the list using two specialities and taking the top two elements of the filtered elements:
let filter: MeetingDoctorsFilterType = MeetingDoctorsFilter(profiles: [.customerCare, .commercial], take: 2)
let result: MeetingDoctorsResult<UINavigationController> = MeetingDoctor.messengerViewController(with: filter) { (contentSize: CGSize) in
// do some stuff to resize layout
}
if let controller: UITableViewController = result.value?.viewControllers.first as? UITableViewController {
// Update the view with the controller
}
Filter - Medical
In order to filter the list of contacts to only show medical professionals, we must use the previous function passing a MeetingDoctorsFilter:
let filter: MeetingDoctorsFilterType = MeetingDoctorsFilter(profiles: [.veterinary, .animalNutrition, .ethology], excludeRoles: true)
let result: MeetingDoctorsResult<UINavigationController> = MeetingDoctor.messengerViewController(with: filter) { (contentSize: CGSize) in
// do some stuff to resize layout
}
if let controller: UITableViewController = result.value?.viewControllers.first as? UITableViewController {
// Update the view with the controller
}
Filter - Veterinary
In order to filter the list of contacts to only show veterinary professionals, we must use the previous function passing a MeetingDoctorsFilter:
let filter: MeetingDoctorsFilterType = MeetingDoctorsFilter(profiles: [.veterinary, .animalNutrition, .ethology], excludeRoles: false)
let result: MeetingDoctorsResult<UINavigationController> = MeetingDoctor.messengerViewController(with: filter) { (contentSize: CGSize) in
// do some stuff to resize layout
}
if let controller: UITableViewController = result.value?.viewControllers.first as? UITableViewController {
// Update the view with the controller
}